This is the multi-page printable view of this section. Click here to print.
Reference
1 - Horreum Clients
Starting from version 0.13
, Horreum comes with some autogenerated clients out of the box that can be used to interact with
the Horreum server programmatically.
Right now there are two clients, one for Python
and the other one for Go
.
Both clients are autogenerated using Kiota openapi generator, and at the moment of writing the autogenerated client is directly exposed
through the HorreumClient
instance. Therefore, the interaction with the raw client follows the Kiota experience, more details can be found in the Kiota documentation.
Prerequisites
In the below sections you can find some usage examples for both clients, here the prerequisites to properly running below examples:
- You must have Horreum server up and running (and accessible). You can find more details on how to setup Horreum server in the Get Started section.
- Generate an API key following these instructions. Then replace
HUSR_00000000_0000_0000_0000_000000000000
with your generated key.
Python Client
Install the latest Horreum client
pip install horreum
Import required Python libraries
import asyncio
asyncio
is required because the client leverages it to perform async requests to the server.
from horreum import new_horreum_client, ClientConfiguration, AuthMethod, HorreumCredentials
new_horreum_client
utility function to setup theHorreumClient
instance
Initialize the Horreum client
client = await new_horreum_client(
base_url="http://localhost:8080",
credentials=HorreumCredentials(
apikey="HUSR_00000000_0000_0000_0000_000000000000"
),
client_config=ClientConfiguration(
auth_method=AuthMethod.API_KEY
)
)
Now let’s start playing with it
# sync call
print("Client version:", client.version())
# async call
print("Server version:", (await client.raw_client.api.config.version.get()).version)
As you might have noticed, the Kiota api follows the REST url pattern. The previous async call was the equivalent of doing
curl -X 'GET' 'http://localhost:8080/api/config/version'
. The URL pattern api/config/version
is replaced by dot notation
api.config.version.<HTTP_METHOD>
.
Golang Client
Install the latest Horreum client
go get github.com/hyperfoil/horreum-client-golang
Import required Go libraries
import (
"github.com/hyperfoil/horreum-client-golang/pkg/horreum"
)
Define username and password
var (
apiKey = "HUSR_00000000_0000_0000_0000_000000000000"
)
Initialize the Horreum client
client, err := horreum.NewHorreumClient("http://localhost:8080",
&horreum.HorreumCredentials{
ApiKey: &apiKey,
}, &horreum.ClientConfiguration{
AuthMethod: horreum.API_KEY,
})
if err != nil {
log.Fatalf("error creating Horreum client: %s", err.Error())
}
Now let’s start playing with it
// sync call
fmt.Printf("Server version: %s\n", *v.GetVersion())
// async call
v, err := client.RawClient.Api().Config().Version().Get(context.Background(), nil)
if err != nil {
log.Fatalf("error getting server version: %s", err.Error())
os.Exit(1)
}
Similarly to the Python example, the previous async call was the equivalent of doing
curl -X 'GET' 'http://localhost:8080/api/config/version'
. The URL pattern api/config/version
is replaced by method chain invocation
api().config().version().<HTTP_METHOD>
. Here you need to provide your own context
to the actual HTTP method call.
2 - Webhook Reference
3 - API Filter Queries
Some APIs provide the ability to filter results when HTTP API response content type is application-json
. The API Filter Query
operates on the server side to reduce payload size.
API operations that support Filter Query provide an HTTP request parameter named query. The value of the query parameter is a JSONPath
expression. Any syntax errors in the JSONPath
will cause the operation to fail with details of the syntax issue.
To use the Filter Query with an operation that returns a Run data can be done as follows.
curl -s 'http://localhost:8080/api/sql/1/queryrun?query=$.*&array=true' -H 'content-type: application/json' -H "X-Horreum-API-Key: $API_KEY"
{
"valid": true,
"jsonpath": "$.*",
"errorCode": 0,
"sqlState": null,
"reason": null,
"sql": null,
"value": "[\"urn:acme:benchmark:0.1\", [{\"test\": \"Foo\", \"duration\": 10, \"requests\": 123}, {\"test\": \"Bar\", \"duration\": 20, \"requests\": 456}], \"defec8eddeadbeafcafebabeb16b00b5\", \"This gets lost by the transformer\"]}"
}
The response contains an enclosing JSON object that is common for Filter Query operations. The value property contains an array. The elements of the array contain the values of properties in the uploaded Run JSON. Note the additional HTTP request query parameter named array. Setting array is necessary to return dynamically sized results.
To filter the above API operation to retrieve only the results property of the uploaded Run object the query parameter is defined as $.results
curl -s 'http://localhost:8080/api/sql/1/queryrun?query=$.results&array=true' -H 'content-type: application/json' -H "X-Horreum-API-Key: $API_KEY"
{
"valid": true,
"jsonpath": "$.results",
"errorCode": 0,
"sqlState": null,
"reason": null,
"sql": null,
"value": "[[{\"test\": \"Foo\", \"duration\": 10, \"requests\": 123}, {\"test\": \"Bar\", \"duration\": 20, \"requests\": 456}]]"
}
The response JSON object this time contains the results property value JSON.
4 - Troubleshooting Functions
Troubleshooting Functions and Combination Function
Creating Scheam Labels
is one of the key capabilities that enables working with loosely defined data. Using a JSON Validation Schema
provides useful guarentees data in an uploaded Run
JSON conforms to expected data types. A Validation Schema
is optional but when not used makes the handling of metrics unreliable as data types may change.
To troubleshoot use of JavaScript Function
the following techniques can be used
- The
typeof
function to discover the data-type of theExtractor
variable, Object property or Array element in theLabel
Combination Function
, using the Test label calculation button to reveal the JavaScript data-type
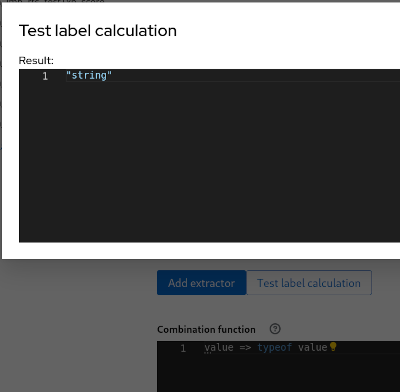
Test label calculation
- View the
Labels calculation Log
window and display INFO or DEBUG log level, this reveals syntax errors when the function is executed
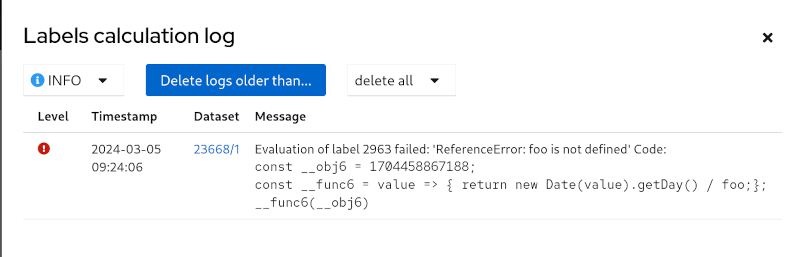
Logged in user, Labels Calculation Log
- Use the Show label values button, provides a quick visual check of resulting values for the
Run
Dataset
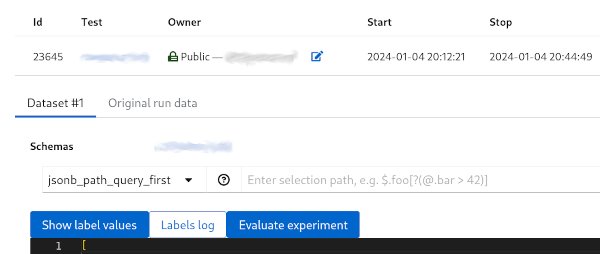
Run Dataset view
Effective label values
window reveals the anticipated value or error text which can be due to the following:
- Nan - Numerical expression involves a data-type that is incompatible
- (undefined) - Incorrect JSONPath field value used in the
Label
Extractor
- empty - Absence of any value in the uploaded
Run
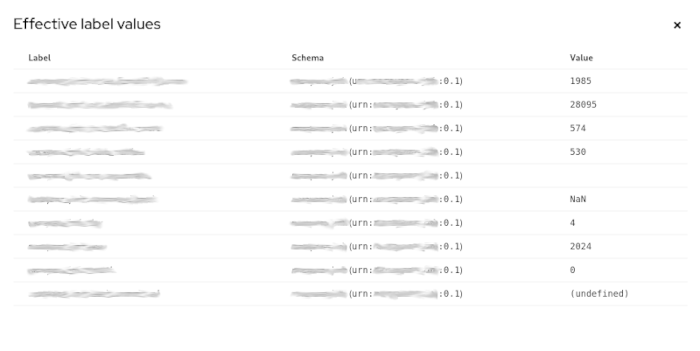
Effective label values